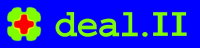 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_fe_dgq_h
17 #define dealii_fe_dgq_h
30 template <
int dim,
int spacedim>
111 template <
int dim,
int spacedim = dim>
121 FE_DGQ(
const unsigned int p);
172 const unsigned int subface,
194 const unsigned int child,
221 const unsigned int child,
248 virtual std::vector<std::pair<unsigned int, unsigned int>>
259 virtual std::vector<std::pair<unsigned int, unsigned int>>
270 virtual std::vector<std::pair<unsigned int, unsigned int>>
290 const unsigned int codim = 0)
const override final;
302 const unsigned int face_index)
const override;
308 virtual std::pair<Table<2, bool>, std::vector<unsigned int>>
321 std::vector<double> & nodal_values)
const override;
323 virtual std::unique_ptr<FiniteElement<dim, spacedim>>
324 clone()
const override;
344 static std::vector<unsigned int>
365 const char direction)
const;
373 template <
int dim1,
int spacedim1>
377 template <
int dim1,
int spacedim1>
399 template <
int dim,
int spacedim = dim>
429 std::vector<double> & nodal_values)
const override;
430 virtual std::unique_ptr<FiniteElement<dim, spacedim>>
431 clone()
const override;
446 template <
int dim,
int spacedim = dim>
461 virtual std::pair<Table<2, bool>, std::vector<unsigned int>>
473 virtual std::unique_ptr<FiniteElement<dim, spacedim>>
474 clone()
const override;
497 template <
int dim,
int spacedim = dim>
516 virtual std::unique_ptr<FiniteElement<dim, spacedim>>
517 clone()
const override;
virtual std::string get_name() const override
virtual bool has_support_on_face(const unsigned int shape_index, const unsigned int face_index) const override
virtual const FullMatrix< double > & get_restriction_matrix(const unsigned int child, const RefinementCase< dim > &refinement_case=RefinementCase< dim >::isotropic_refinement) const override
void rotate_indices(std::vector< unsigned int > &indices, const char direction) const
virtual std::unique_ptr< FiniteElement< dim, spacedim > > clone() const override
virtual std::string get_name() const override
const unsigned int degree
virtual std::vector< std::pair< unsigned int, unsigned int > > hp_line_dof_identities(const FiniteElement< dim, spacedim > &fe_other) const override
FE_DGQArbitraryNodes(const Quadrature< 1 > &points)
virtual std::unique_ptr< FiniteElement< dim, spacedim > > clone() const override
virtual std::unique_ptr< FiniteElement< dim, spacedim > > clone() const override
#define DEAL_II_NAMESPACE_OPEN
virtual std::string get_name() const override
@ matrix
Contents is actually a matrix.
virtual void get_subface_interpolation_matrix(const FiniteElement< dim, spacedim > &source, const unsigned int subface, FullMatrix< double > &matrix) const override
virtual std::vector< std::pair< unsigned int, unsigned int > > hp_vertex_dof_identities(const FiniteElement< dim, spacedim > &fe_other) const override
virtual FiniteElementDomination::Domination compare_for_domination(const FiniteElement< dim, spacedim > &fe_other, const unsigned int codim=0) const override final
virtual std::pair< Table< 2, bool >, std::vector< unsigned int > > get_constant_modes() const override
virtual const FullMatrix< double > & get_prolongation_matrix(const unsigned int child, const RefinementCase< dim > &refinement_case=RefinementCase< dim >::isotropic_refinement) const override
virtual void convert_generalized_support_point_values_to_dof_values(const std::vector< Vector< double >> &support_point_values, std::vector< double > &nodal_values) const override
virtual void get_face_interpolation_matrix(const FiniteElement< dim, spacedim > &source, FullMatrix< double > &matrix) const override
virtual std::string get_name() const override
static std::vector< unsigned int > get_dpo_vector(const unsigned int degree)
FE_DGQHermite(const unsigned int degree)
virtual void get_interpolation_matrix(const FiniteElement< dim, spacedim > &source, FullMatrix< double > &matrix) const override
FE_DGQLegendre(const unsigned int degree)
virtual std::unique_ptr< FiniteElement< dim, spacedim > > clone() const override
#define DEAL_II_NAMESPACE_CLOSE
virtual bool hp_constraints_are_implemented() const override
virtual void convert_generalized_support_point_values_to_dof_values(const std::vector< Vector< double >> &support_point_values, std::vector< double > &nodal_values) const override
virtual std::pair< Table< 2, bool >, std::vector< unsigned int > > get_constant_modes() const override
virtual std::vector< std::pair< unsigned int, unsigned int > > hp_quad_dof_identities(const FiniteElement< dim, spacedim > &fe_other) const override