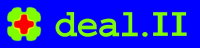 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_dof_handler_policy_h
17 # define dealii_dof_handler_policy_h
43 namespace DoFHandlerImplementation
60 template <
int dim,
int spacedim>
85 virtual std::vector<NumberCache>
95 const std::vector<types::global_dof_index> &new_numbers)
const = 0;
107 const unsigned int level,
108 const std::vector<types::global_dof_index> &new_numbers)
const = 0;
116 template <
class DoFHandlerType>
118 DoFHandlerType::space_dimension>
133 virtual std::vector<NumberCache>
138 renumber_dofs(
const std::vector<types::global_dof_index> &new_numbers)
144 const std::vector<types::global_dof_index>
145 &new_numbers)
const override;
160 template <
class DoFHandlerType>
162 DoFHandlerType::space_dimension>
186 virtual std::vector<NumberCache>
199 renumber_dofs(
const std::vector<types::global_dof_index> &new_numbers)
205 const std::vector<types::global_dof_index>
206 &new_numbers)
const override;
220 template <
class DoFHandlerType>
222 :
public PolicyBase<DoFHandlerType::dimension,
223 DoFHandlerType::space_dimension>
238 virtual std::vector<NumberCache>
243 renumber_dofs(
const std::vector<types::global_dof_index> &new_numbers)
249 const std::vector<types::global_dof_index>
250 &new_numbers)
const override;
SmartPointer< DoFHandlerType > dof_handler
virtual NumberCache distribute_dofs() const override
ParallelDistributed(DoFHandlerType &dof_handler)
virtual ~PolicyBase()=default
virtual NumberCache renumber_dofs(const std::vector< types::global_dof_index > &new_numbers) const override
virtual NumberCache distribute_dofs() const override
virtual NumberCache distribute_dofs() const =0
virtual NumberCache distribute_dofs() const override
virtual NumberCache renumber_mg_dofs(const unsigned int level, const std::vector< types::global_dof_index > &new_numbers) const override
virtual std::vector< NumberCache > distribute_mg_dofs() const override
Sequential(DoFHandlerType &dof_handler)
virtual NumberCache renumber_mg_dofs(const unsigned int level, const std::vector< types::global_dof_index > &new_numbers) const =0
virtual std::vector< NumberCache > distribute_mg_dofs() const override
#define DEAL_II_NAMESPACE_OPEN
virtual NumberCache renumber_dofs(const std::vector< types::global_dof_index > &new_numbers) const override
virtual NumberCache renumber_dofs(const std::vector< types::global_dof_index > &new_numbers) const override
virtual NumberCache renumber_mg_dofs(const unsigned int level, const std::vector< types::global_dof_index > &new_numbers) const override
ParallelShared(DoFHandlerType &dof_handler)
virtual NumberCache renumber_mg_dofs(const unsigned int level, const std::vector< types::global_dof_index > &new_numbers) const override
#define DEAL_II_NAMESPACE_CLOSE
virtual std::vector< NumberCache > distribute_mg_dofs() const =0
SmartPointer< DoFHandlerType > dof_handler
SmartPointer< DoFHandlerType > dof_handler
virtual NumberCache renumber_dofs(const std::vector< types::global_dof_index > &new_numbers) const =0
virtual std::vector< NumberCache > distribute_mg_dofs() const override