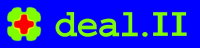 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_diagonal_matrix_h
17 #define dealii_diagonal_matrix_h
49 template <
typename VectorType = Vector<
double>>
153 template <
typename number2>
158 const number2 * values,
159 const bool elide_zero_values =
true,
160 const bool col_indices_are_sorted =
false);
228 template <
typename VectorType>
235 template <
typename VectorType>
244 template <
typename VectorType>
248 return diagonal.memory_consumption();
253 template <
typename VectorType>
262 template <
typename VectorType>
271 template <
typename VectorType>
280 template <
typename VectorType>
289 template <
typename VectorType>
298 template <
typename VectorType>
307 template <
typename VectorType>
316 template <
typename VectorType>
317 typename VectorType::value_type
328 template <
typename VectorType>
329 typename VectorType::value_type &
339 template <
typename VectorType>
340 template <
typename number2>
345 const number2 * values,
350 if (col_indices[i] == row)
356 template <
typename VectorType>
368 template <
typename VectorType>
378 template <
typename VectorType>
387 template <
typename VectorType>
399 template <
typename VectorType>
value_type operator()(const size_type i, const size_type j) const
@ diagonal
Matrix is diagonal.
void initialize_dof_vector(VectorType &dst) const
typename LinearAlgebra::distributed::Vector< double > ::size_type size_type
void add(const size_type row, const size_type n_cols, const size_type *col_indices, const number2 *values, const bool elide_zero_values=true, const bool col_indices_are_sorted=false)
void compress(VectorOperation::values operation)
static ::ExceptionBase & ExcIndexRange(int arg1, int arg2, int arg3)
void Tvmult_add(VectorType &dst, const VectorType &src) const
#define DEAL_II_NAMESPACE_OPEN
typename LinearAlgebra::distributed::Vector< double > ::value_type value_type
VectorType & get_vector()
types::global_dof_index size_type
void reinit(const VectorType &vec)
void vmult_add(VectorType &dst, const VectorType &src) const
#define Assert(cond, exc)
void Tvmult(VectorType &dst, const VectorType &src) const
#define DEAL_II_NAMESPACE_CLOSE
void vmult(VectorType &dst, const VectorType &src) const
std::size_t memory_consumption() const