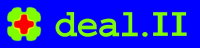 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_data_out_stack_h
17 #define dealii_data_out_stack_h
36 template <
int dim,
int spacedim>
208 template <
typename number>
230 template <
typename number>
233 const std::vector<std::string> &names);
275 <<
"The data vector for which the first component has the name " << arg1
276 <<
" has not been added before.");
281 "You cannot start a new time/parameter step before calling "
282 "finish_parameter_value() on the previous step.");
288 "You cannot declare additional vectors after already calling "
289 "build_patches(). All data vectors need to be declared "
290 "before you call this function the first time.");
296 <<
"You tried to declare a component of a data vector with "
297 <<
"the name <" << arg1
298 <<
">, but that name is already used.");
323 std::vector<::DataOutBase::Patch<dim + 1, dim + 1>>
patches;
364 virtual const std::vector<::DataOutBase::Patch<dim + 1, dim + 1>> &
372 virtual std::vector<std::string>
#define DeclExceptionMsg(Exception, defaulttext)
static ::ExceptionBase & ExcNameAlreadyUsed(std::string arg1)
virtual std::vector< std::string > get_dataset_names() const override
static ::ExceptionBase & ExcDataNotCleared()
std::vector< std::string > names
virtual const std::vector<::DataOutBase::Patch< dim+1, dim+1 > > & get_patches() const override
void attach_dof_handler(const DoFHandlerType &dof_handler)
std::vector< DataVector > dof_data
#define DEAL_II_NAMESPACE_OPEN
#define DeclException1(Exception1, type1, outsequence)
SmartPointer< const DoFHandlerType, DataOutStack< dim, spacedim, DoFHandlerType > > dof_handler
std::size_t memory_consumption() const
std::size_t memory_consumption() const
void declare_data_vector(const std::string &name, const VectorType vector_type)
std::vector<::DataOutBase::Patch< dim+1, dim+1 > > patches
virtual ~DataOutStack() override=default
void new_parameter_value(const double parameter_value, const double parameter_step)
void add_data_vector(const Vector< number > &vec, const std::string &name)
static ::ExceptionBase & ExcVectorNotDeclared(std::string arg1)
void finish_parameter_value()
#define DEAL_II_NAMESPACE_CLOSE
void build_patches(const unsigned int n_subdivisions=0)
static ::ExceptionBase & ExcDataAlreadyAdded()
std::vector< DataVector > cell_data