Loading [MathJax]/extensions/TeX/AMSsymbols.js
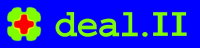 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_constrained_linear_operator_h
17 #define dealii_constrained_linear_operator_h
64 template <
typename Range,
typename Domain,
typename Payload>
72 return_op.
vmult_add = [&constraints](Range &v,
const Domain &u) {
74 ::
ExcMessage(
"The domain and range vectors must be different "
75 "storage locations"));
81 const auto &locally_owned_elements = v.locally_owned_elements();
82 for (
const auto &line : constraints.
get_lines())
84 const auto i = line.index;
85 if (locally_owned_elements.is_element(i))
88 const auto &entries = line.entries;
91 const auto pos = entries[j].first;
92 v(i) += u(pos) * entries[j].second;
100 return_op.
Tvmult_add = [&constraints](Domain &v,
const Range &u) {
102 ::
ExcMessage(
"The domain and range vectors must be different "
103 "storage locations"));
109 const auto &locally_owned_elements = v.locally_owned_elements();
110 for (
const auto &line : constraints.
get_lines())
112 const auto i = line.index;
114 if (locally_owned_elements.is_element(i))
119 const auto &entries = line.entries;
122 const auto pos = entries[j].first;
123 if (locally_owned_elements.is_element(pos))
124 v(pos) += u(i) * entries[j].second;
132 const auto vmult_add = return_op.
vmult_add;
133 return_op.
vmult = [vmult_add](Range &v,
const Domain &u) {
140 return_op.
Tvmult = [Tvmult_add](Domain &v,
const Range &u) {
160 template <
typename Range,
typename Domain,
typename Payload>
168 return_op.
vmult_add = [&constraints](Range &v,
const Domain &u) {
169 const auto &locally_owned_elements = v.locally_owned_elements();
170 for (
const auto &line : constraints.
get_lines())
172 const auto i = line.index;
173 if (locally_owned_elements.is_element(i))
182 return_op.
Tvmult_add = [&constraints](Domain &v,
const Range &u) {
183 const auto &locally_owned_elements = v.locally_owned_elements();
184 for (
const auto &line : constraints.
get_lines())
186 const auto i = line.index;
187 if (locally_owned_elements.is_element(i))
197 const auto vmult_add = return_op.
vmult_add;
198 return_op.
vmult = [vmult_add](Range &v,
const Domain &u) {
205 return_op.
Tvmult = [Tvmult_add](Domain &v,
const Range &u) {
251 template <
typename Range,
typename Domain,
typename Payload>
260 return Ct * linop *
C + Id_c;
298 template <
typename Range,
typename Domain,
typename Payload>
303 const Range & right_hand_side)
309 return_comp.
apply_add = [&constraints, &linop, &right_hand_side](Range &v) {
315 linop.reinit_domain_vector(*k,
false);
318 v += Ct * (right_hand_side - linop * *k);
322 const auto apply_add = return_comp.
apply_add;
323 return_comp.
apply = [apply_add](Range &v) {
PackagedOperation< Range > constrained_right_hand_side(const AffineConstraints< typename Range::value_type > &constraints, const LinearOperator< Range, Domain, Payload > &linop, const Range &right_hand_side)
LinearOperator< Range, Domain, Payload > project_to_constrained_linear_operator(const AffineConstraints< typename Range::value_type > &constraints, const LinearOperator< Range, Domain, Payload > &exemplar)
static bool equal(const T *p1, const T *p2)
std::function< void(Range &v, bool omit_zeroing_entries)> reinit_vector
SymmetricTensor< 2, dim, Number > C(const Tensor< 2, dim, Number > &F)
std::function< void(Range &v, const Domain &u)> vmult_add
std::function< void(Range &v, const Domain &u)> vmult
const LineRange get_lines() const
std::function< void(Range &v, bool omit_zeroing_entries)> reinit_range_vector
std::function< void(Domain &v, const Range &u)> Tvmult_add
static ::ExceptionBase & ExcMessage(std::string arg1)
LinearOperator< Range, Domain, Payload > distribute_constraints_linear_operator(const AffineConstraints< typename Range::value_type > &constraints, const LinearOperator< Range, Domain, Payload > &exemplar)
std::function< void(Range &v)> apply_add
#define DEAL_II_NAMESPACE_OPEN
std::function< void(Domain &v, const Range &u)> Tvmult
void distribute(VectorType &vec) const
#define Assert(cond, exc)
LinearOperator< Domain, Range, Payload > transpose_operator(const LinearOperator< Range, Domain, Payload > &op)
#define DEAL_II_NAMESPACE_CLOSE
std::function< void(Range &v)> apply
LinearOperator< Range, Domain, Payload > constrained_linear_operator(const AffineConstraints< typename Range::value_type > &constraints, const LinearOperator< Range, Domain, Payload > &linop)