Loading [MathJax]/extensions/TeX/AMSsymbols.js
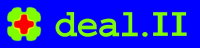 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_vector_tools_project_h
17 #define dealii_vector_tools_project_h
27 template <
typename number>
29 template <
int dim,
int spacedim>
31 template <
int dim,
typename Number>
33 template <
int dim,
int spacedim>
35 template <
int dim,
typename number,
typename VectorizedArrayType>
41 template <
typename Number, std::
size_t w
idth>
45 template <
int dim,
int spacedim>
47 template <
int dim,
int spacedim>
48 class MappingCollection;
141 template <
int dim,
typename VectorType,
int spacedim>
149 const bool enforce_zero_boundary =
false,
153 const bool project_to_boundary_first =
false);
159 template <
int dim,
typename VectorType,
int spacedim>
166 const bool enforce_zero_boundary =
false,
170 const bool project_to_boundary_first =
false);
176 template <
int dim,
typename VectorType,
int spacedim>
184 const bool enforce_zero_boundary =
false,
187 const bool project_to_boundary_first =
false);
193 template <
int dim,
typename VectorType,
int spacedim>
200 const bool enforce_zero_boundary =
false,
203 const bool project_to_boundary_first =
false);
229 template <
int dim,
typename VectorType,
int spacedim>
235 const std::function<
typename VectorType::value_type(
237 const unsigned int)> & func,
268 template <
int dim,
typename VectorType>
273 typename VectorType::value_type,
276 const unsigned int n_q_points_1d,
279 const unsigned int)> & func,
281 const unsigned int fe_component = 0);
287 template <
int dim,
typename VectorType>
292 typename VectorType::value_type,
297 const unsigned int)> & func,
299 const unsigned int fe_component = 0);
307 #endif // dealii_vector_tools_project_h
typename ActiveSelector::active_cell_iterator active_cell_iterator
Abstract base class for mapping classes.
#define DEAL_II_NAMESPACE_OPEN
#define DEAL_II_NAMESPACE_CLOSE