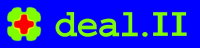 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_mesh_worker_vector_selector_h
17 #define dealii_mesh_worker_vector_selector_h
64 add(
const std::string &name,
65 const bool values =
true,
66 const bool gradients =
false,
67 const bool hessians =
false);
154 template <
class StreamType,
typename DATA>
161 template <
class StreamType>
163 print(StreamType &s)
const;
195 template <
int dim,
int spacedim = dim,
typename Number =
double>
263 fill(std::vector<std::vector<std::vector<Number>>> &values,
269 const std::vector<types::global_dof_index> &index,
270 const unsigned int component,
271 const unsigned int n_comp,
272 const unsigned int start,
273 const unsigned int size)
const;
282 mg_fill(std::vector<std::vector<std::vector<Number>>> &values,
288 const unsigned int level,
289 const std::vector<types::global_dof_index> &index,
290 const unsigned int component,
291 const unsigned int n_comp,
292 const unsigned int start,
293 const unsigned int size)
const;
308 template <
typename VectorType,
int dim,
int spacedim = dim>
310 :
public VectorDataBase<dim, spacedim, typename VectorType::value_type>
340 fill(std::vector<std::vector<std::vector<typename VectorType::value_type>>>
342 std::vector<std::vector<
345 std::vector<std::vector<
349 const std::vector<types::global_dof_index> &index,
350 const unsigned int component,
351 const unsigned int n_comp,
352 const unsigned int start,
353 const unsigned int size)
const override;
357 std::vector<std::vector<std::vector<typename VectorType::value_type>>>
359 std::vector<std::vector<
362 std::vector<std::vector<
366 const unsigned int level,
367 const std::vector<types::global_dof_index> &index,
368 const unsigned int component,
369 const unsigned int n_comp,
370 const unsigned int start,
371 const unsigned int size)
const override;
389 template <
typename VectorType,
int dim,
int spacedim = dim>
426 const bool gradients,
528 template <
class StreamType>
533 <<
" hessians: " <<
n_hessians() << std::endl;
537 template <
class StreamType,
typename DATA>
542 for (
unsigned int i = 0; i <
n_values(); ++i)
544 s << std::endl <<
"gradients:";
547 s << std::endl <<
"hessians: ";
548 for (
unsigned int i = 0; i <
n_hessians(); ++i)
557 return sizeof(*this);
virtual void fill(std::vector< std::vector< std::vector< typename VectorType::value_type >>> &values, std::vector< std::vector< std::vector< Tensor< 1, spacedim, typename VectorType::value_type >>>> &gradients, std::vector< std::vector< std::vector< Tensor< 2, spacedim, typename VectorType::value_type >>>> &hessians, const FEValuesBase< dim, spacedim > &fe, const std::vector< types::global_dof_index > &index, const unsigned int component, const unsigned int n_comp, const unsigned int start, const unsigned int size) const override
unsigned int hessian_index(const unsigned int i) const
void initialize(const AnyData &data)
std::size_t memory_consumption() const
void initialize(const AnyData &)
unsigned int gradient_index(const unsigned int i) const
unsigned int size() const
virtual void fill(std::vector< std::vector< std::vector< Number >>> &values, std::vector< std::vector< std::vector< Tensor< 1, spacedim, Number >>>> &gradients, std::vector< std::vector< std::vector< Tensor< 2, spacedim, Number >>>> &hessians, const FEValuesBase< dim, spacedim > &fe, const std::vector< types::global_dof_index > &index, const unsigned int component, const unsigned int n_comp, const unsigned int start, const unsigned int size) const
const std::string & name(const unsigned int i) const
Name of object at index.
void add(const std::string &name)
void initialize(const AnyData &)
bool has_hessians() const
NamedSelection hessian_selection
std::size_t memory_consumption() const
unsigned int n_gradients() const
virtual void mg_fill(std::vector< std::vector< std::vector< Number >>> &values, std::vector< std::vector< std::vector< Tensor< 1, spacedim, Number >>>> &gradients, std::vector< std::vector< std::vector< Tensor< 2, spacedim, Number >>>> &hessians, const FEValuesBase< dim, spacedim > &fe, const unsigned int level, const std::vector< types::global_dof_index > &index, const unsigned int component, const unsigned int n_comp, const unsigned int start, const unsigned int size) const
unsigned int value_index(const unsigned int i) const
unsigned int n_hessians() const
void print(StreamType &s, const AnyData &v) const
void initialize(const AnyData &)
#define DEAL_II_NAMESPACE_OPEN
NamedSelection value_selection
NamedSelection gradient_selection
bool has_gradients() const
void initialize(const AnyData &)
void add(const std::string &name, const bool values=true, const bool gradients=false, const bool hessians=false)
virtual void mg_fill(std::vector< std::vector< std::vector< typename VectorType::value_type >>> &values, std::vector< std::vector< std::vector< Tensor< 1, spacedim, typename VectorType::value_type >>>> &gradients, std::vector< std::vector< std::vector< Tensor< 2, spacedim, typename VectorType::value_type >>>> &hessians, const FEValuesBase< dim, spacedim > &fe, const unsigned int level, const std::vector< types::global_dof_index > &index, const unsigned int component, const unsigned int n_comp, const unsigned int start, const unsigned int size) const override
virtual ~VectorDataBase() override=default
#define DEAL_II_NAMESPACE_CLOSE
unsigned int n_values() const