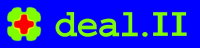 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_time_stepping_h
17 #define dealii_time_stepping_h
95 template <
typename VectorType>
115 evolve_one_time_step(
118 std::vector<std::function<
134 get_status()
const = 0;
145 template <
typename VectorType>
175 std::vector<std::function<
197 & id_minus_tau_J_inverse,
211 std::vector<double>
b;
216 std::vector<double>
c;
221 std::vector<std::vector<double>>
a;
230 template <
typename VectorType>
270 & id_minus_tau_J_inverse,
315 const double delta_t,
317 std::vector<VectorType> &f_stages)
const;
331 template <
typename VectorType>
350 const unsigned int max_it = 100,
375 & id_minus_tau_J_inverse,
420 & id_minus_tau_J_inverse,
424 std::vector<VectorType> &f_stages);
433 & id_minus_tau_J_inverse,
478 template <
typename VectorType>
498 const double min_delta = 1
e-14,
499 const double max_delta = 1e100,
539 & id_minus_tau_J_inverse,
564 const double min_delta,
565 const double max_delta,
598 const double delta_t,
600 std::vector<VectorType> &f_stages);
645 std::vector<double>
b1;
650 std::vector<double>
b2;
double evolve_one_time_step(const std::function< VectorType(const double, const VectorType &)> &f, const std::function< VectorType(const double, const double, const VectorType &)> &id_minus_tau_J_inverse, double t, double delta_t, VectorType &y) override
double evolve_one_time_step(std::vector< std::function< VectorType(const double, const VectorType &)>> &F, std::vector< std::function< VectorType(const double, const double, const VectorType &)>> &J_inverse, double t, double delta_t, VectorType &y) override
Tensor< 2, dim, Number > F(const Tensor< 2, dim, Number > &Grad_u)
~EmbeddedExplicitRungeKutta() override
const Status & get_status() const override
runge_kutta_method method
virtual ~RungeKutta() override=default
void compute_stages(const std::function< VectorType(const double, const VectorType &)> &f, const std::function< VectorType(const double, const double, const VectorType &)> &id_minus_tau_J_inverse, double t, double delta_t, VectorType &y, std::vector< VectorType > &f_stages)
ExplicitRungeKutta()=default
SymmetricTensor< 2, dim, Number > e(const Tensor< 2, dim, Number > &F)
double evolve_one_time_step(const std::function< VectorType(const double, const VectorType &)> &f, const std::function< VectorType(const double, const double, const VectorType &)> &id_minus_tau_J_inverse, double t, double delta_t, VectorType &y) override
void initialize(const runge_kutta_method method) override
void initialize(const runge_kutta_method method) override
std::vector< std::vector< double > > a
runge_kutta_method method
const Status & get_status() const override
#define DEAL_II_NAMESPACE_OPEN
void compute_stages(const std::function< VectorType(const double, const VectorType &)> &f, const double t, const double delta_t, const VectorType &y, std::vector< VectorType > &f_stages) const
void compute_residual(const std::function< VectorType(const double, const VectorType &)> &f, double t, double delta_t, const VectorType &new_y, const VectorType &y, VectorType &tendency, VectorType &residual) const
runge_kutta_method method
void set_newton_solver_parameters(const unsigned int max_it, const double tolerance)
embedded_runge_kutta_time_step exit_delta_t
void initialize(const runge_kutta_method method) override
unsigned int n_iterations
@ RK_CLASSIC_FOURTH_ORDER
virtual void initialize(const runge_kutta_method method)=0
unsigned int n_iterations
ImplicitRungeKutta()=default
EmbeddedExplicitRungeKutta()=default
double evolve_one_time_step(const std::function< VectorType(const double, const VectorType &)> &f, const std::function< VectorType(const double, const double, const VectorType &)> &id_minus_tau_J_inverse, double t, double delta_t, VectorType &y) override
static const unsigned int invalid_unsigned_int
embedded_runge_kutta_time_step
#define DEAL_II_NAMESPACE_CLOSE
void set_time_adaptation_parameters(const double coarsen_param, const double refine_param, const double min_delta, const double max_delta, const double refine_tol, const double coarsen_tol)
void newton_solve(const std::function< void(const VectorType &, VectorType &)> &get_residual, const std::function< VectorType(const VectorType &)> &id_minus_tau_J_inverse, VectorType &y)
const Status & get_status() const override
void compute_stages(const std::function< VectorType(const double, const VectorType &)> &f, const double t, const double delta_t, const VectorType &y, std::vector< VectorType > &f_stages)