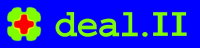 |
Reference documentation for deal.II version 9.2.0
|
\(\newcommand{\dealvcentcolon}{\mathrel{\mathop{:}}}\)
\(\newcommand{\dealcoloneq}{\dealvcentcolon\mathrel{\mkern-1.2mu}=}\)
\(\newcommand{\jump}[1]{\left[\!\left[ #1 \right]\!\right]}\)
\(\newcommand{\average}[1]{\left\{\!\left\{ #1 \right\}\!\right\}}\)
Go to the documentation of this file.
16 #ifndef dealii_fe_dg_vector_h
17 #define dealii_fe_dg_vector_h
54 template <
class PolynomialType,
int dim,
int spacedim = dim>
72 virtual std::unique_ptr<FiniteElement<dim, spacedim>>
73 clone()
const override;
83 const unsigned int face_index)
const override;
95 static std::vector<unsigned int>
147 template <
int dim,
int spacedim = dim>
180 template <
int dim,
int spacedim = dim>
182 :
public FE_DGVector<PolynomialsRaviartThomas<dim>, dim, spacedim>
213 template <
int dim,
int spacedim = dim>
static std::vector< unsigned int > get_dpo_vector(const unsigned int degree)
virtual std::string get_name() const override
virtual std::string get_name() const override
Table< 3, double > interior_weights
virtual std::string get_name() const override
FE_DGVector(const unsigned int p, MappingKind m)
const unsigned int degree
std::vector< std::vector< Tensor< 1, dim > > > shape_values
FE_DGNedelec(const unsigned int p)
virtual std::size_t memory_consumption() const override
virtual std::string get_name() const override
#define DEAL_II_NAMESPACE_OPEN
virtual bool has_support_on_face(const unsigned int shape_index, const unsigned int face_index) const override
FE_DGBDM(const unsigned int p)
FE_DGRaviartThomas(const unsigned int p)
virtual std::unique_ptr< FiniteElement< dim, spacedim > > clone() const override
std::vector< std::vector< Tensor< 2, dim > > > shape_gradients
#define DEAL_II_NAMESPACE_CLOSE